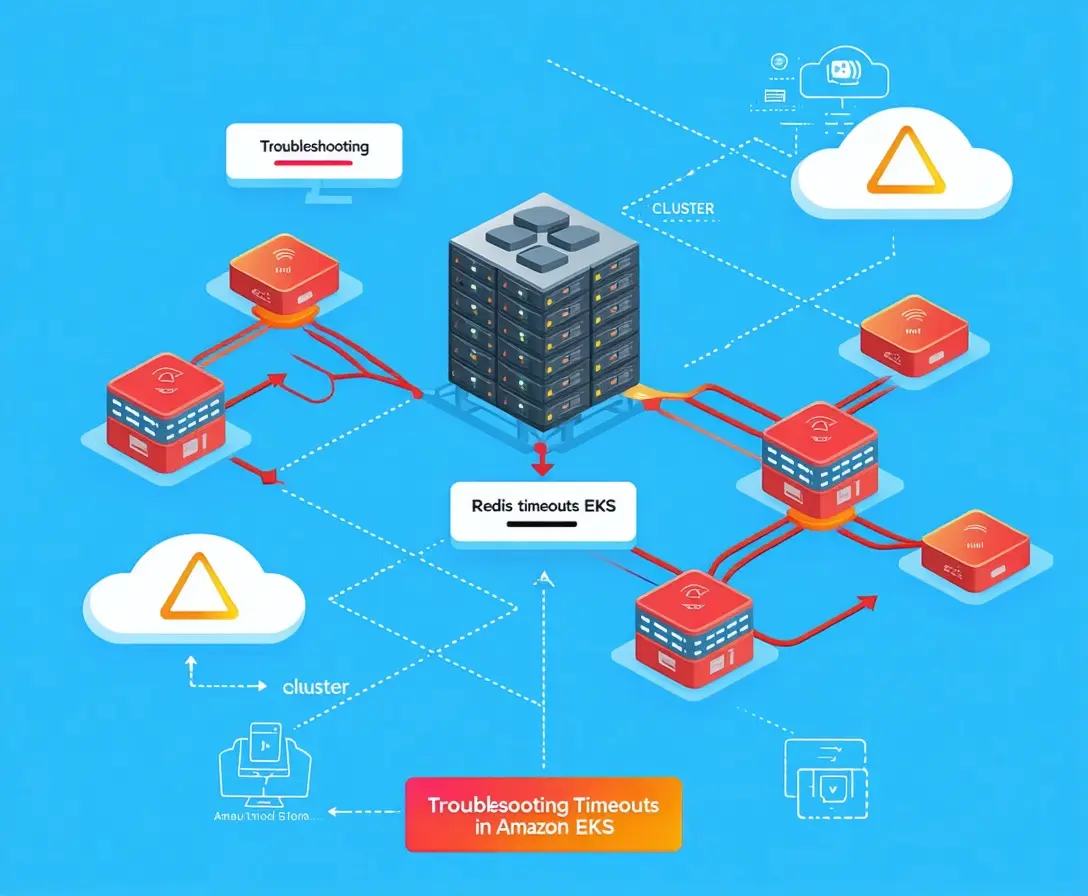
Troubleshooting Connectivity and Redis Timeouts in Amazon EKS Environments
Redis has become a cornerstone technology in modern application architectures, serving as a versatile in-memory data store for caching, session management, pub/sub messaging, and more. When deployed alongside containerized applications in Amazon Elastic Kubernetes Service (EKS), teams frequently encounter connectivity challenges that can be difficult to diagnose and resolve. The article explores various connectivity problems such as intermittent timeouts during high traffic, connection issues during cluster scaling, inconsistent performance across namespaces, and unexpected connection drops.
Introduction
These connectivity issues and timeouts are particularly frustrating because they often manifest intermittently and under load conditions that are difficult to replicate in development environments. Teams migrating to EKS or scaling their Redis usage commonly struggle with:
- Intermittent timeouts that appear during high-traffic periods
- Connection problems after cluster scaling events
- Inconsistent performance across different Kubernetes namespaces
- Unexpected connection drops between application pods and Redis endpoints
The impact of these issues ranges from degraded user experience to complete service outages, making reliable Redis connectivity a critical operational concern. This article aims to provide a comprehensive guide for diagnosing, troubleshooting, and resolving Redis connectivity challenges in EKS environments.
Common Causes of Redis Timeouts in Kubernetes Environments
Before diving into specific troubleshooting techniques, it’s important to understand the primary causes of Redis timeouts in containerized environments:
Connection Pool Exhaustion
Modern applications typically use connection pooling to efficiently manage Redis connections. In Kubernetes, as pods scale dynamically, connection pools can rapidly multiply:
- Each pod might maintain its own connection pool (often 5-50 connections)
- During scaling events, the total connections can surge beyond Redis limits
- Default connection limits in Redis (10,000 connections1) may seem high, but performance often degrades well before reaching this limit
Example application log showing connection pool exhaustion:
2023-08-12T14:25:18.456Z ERROR [app-service] - Redis connection error: JedisPoolExhaustedException: Could not get a resource from the pool
Network Latency and Instability
Kubernetes adds additional network layers that can introduce latency:
- Container Network Interface (CNI) routing overhead
- Cross-Availability Zone traffic if pods and Redis are in different AZs
- DNS resolution delays or failures
- Pod evictions causing connection reestablishment storms
Misaligned Timeout Configurations
Timeout inconsistencies across the technology stack frequently cause problems:
- Redis server has its own timeout settings
- Client libraries maintain separate timeouts for connections and operations
- Kubernetes readiness/liveness probe timeouts add another layer
- Load balancers or proxies in front of Redis may have their own timeout settings
Resource Constraints
Resource limitations on either Redis or the client side can manifest as connectivity issues:
- Redis server under memory pressure triggering evictions or slow operations
- CPU constraints on application pods causing slow processing of Redis responses
- Network throttling at the node or pod level
Network Connectivity Troubleshooting Between EKS Pods and Redis
When facing connectivity issues, a systematic approach to network troubleshooting is essential. This involves methodically testing each layer of connectivity, from basic TCP/IP access to application-level Redis commands, while monitoring system metrics and logs to identify potential bottlenecks or failure points. By following a structured troubleshooting process, you can more quickly isolate and resolve Redis connectivity problems in your EKS environments.
Basic Connectivity Tests
Start with fundamental connectivity verification:
# Create a debugging pod in the same namespace (In some cases you may need to deploy to a specific node)
kubectl run redis-debug --rm -it --image=redis:alpine -- sh
# Test basic connectivity
nc -zv my-redis-master.xxxx.ng.0001.use1.cache.amazonaws.com 6379
# Test with Redis CLI
redis-cli -h my-redis-master.xxxx.ng.0001.use1.cache.amazonaws.com ping
# Check DNS resolution
nslookup my-redis-master.xxxx.ng.0001.use1.cache.amazonaws.com
# Examine TCP connection details
tcpdump -i any port 6379 -vv
Security Group Configuration
For ElastiCache Redis or EC2-hosted Redis, security groups are a common source of connectivity issues:
# Identify security groups assigned to your EKS nodes
NODE_SG=$(aws eks describe-nodegroup --cluster-name my-cluster \
--nodegroup-name my-nodegroup --query 'nodegroup.resources.remoteAccessSecurityGroup' \
--output text)
# Ensure the Redis security group allows traffic from node security group
aws ec2 authorize-security-group-ingress \
--group-id sg-0123456789abcdef0 \ # Redis security group
--source-group $NODE_SG \
--protocol tcp \
--port 6379
Network Policy Analysis
If you’re using Kubernetes Network Policies, verify they’re not blocking Redis traffic:
apiVersion: networking.k8s.io/v1
kind: NetworkPolicy
metadata:
name: allow-redis-egress
namespace: application
spec:
podSelector:
matchLabels:
app: my-application
policyTypes:
- Egress
egress:
- to:
- ipBlock:
cidr: 10.0.0.0/16 # Subnet containing Redis
ports:
- protocol: TCP
port: 6379
VPC and Subnet Routing
For complex VPC configurations, verify routing between EKS subnets and Redis:
# Check if pods are in the expected subnets
kubectl get pods -o wide
# Verify route tables associated with these subnets
aws ec2 describe-route-tables --filters "Name=association.subnet-id,Values=subnet-12345"
# For Redis in a different VPC, check peering connections
aws ec2 describe-vpc-peering-connections
Configuration Best Practices for Redis Clients in Containerized Environments
Properly configuring Redis clients can dramatically improve reliability in Kubernetes environments.
Connection Pool Sizing
Rather than using default settings, explicitly configure connection pools based on your workload2:
// Java example with Lettuce client
@Bean
public LettuceConnectionFactory redisConnectionFactory() {
LettuceClientConfiguration clientConfig = LettuceClientConfiguration.builder()
.poolConfig(poolConfig())
.commandTimeout(Duration.ofMillis(500))
.shutdownTimeout(Duration.ZERO)
.build();
return new LettuceConnectionFactory(redisStandaloneConfig(), clientConfig);
}
@Bean
public GenericObjectPoolConfig poolConfig() {
GenericObjectPoolConfig config = new GenericObjectPoolConfig();
config.setMaxTotal(20); // Max connections per pod
config.setMaxIdle(10); // Connections to maintain when idle
config.setMinIdle(5); // Minimum idle connections to maintain
config.setTestOnBorrow(true); // Validate connections when borrowed
config.setTestWhileIdle(true); // Periodically test idle connections
config.setMaxWait(Duration.ofMillis(1000)); // Max wait for connection
return config;
}
For Node.js applications:
// Node.js with ioredis
const Redis = require('ioredis');
const redis = new Redis({
host: process.env.REDIS_HOST,
port: 6379,
password: process.env.REDIS_PASSWORD,
db: 0,
maxRetriesPerRequest: 3,
connectTimeout: 1000,
commandTimeout: 500,
retryStrategy(times) {
const delay = Math.min(times * 50, 2000);
return delay;
}
});
Timeouts and Retries
Set timeouts appropriately for your application needs:
# Python with redis-py
import redis
from redis.retry import Retry
retry = Retry(ExponentialBackoff(), 3)
redis_client = redis.Redis(
host=os.environ.get('REDIS_HOST'),
port=6379,
socket_timeout=1.0, # Operation timeout
socket_connect_timeout=1.0, # Connection timeout
retry_on_timeout=True,
retry=retry,
health_check_interval=30 # Verify connections every 30 seconds
)
Graceful Connection Handling During Pod Lifecycle
Properly managing connections during pod startup and shutdown is critical but often overlooked:
// Java Spring Boot example
@PreDestroy
public void cleanupRedisConnections() {
if (redisConnectionFactory instanceof LettuceConnectionFactory) {
((LettuceConnectionFactory) redisConnectionFactory).destroy();
log.info("Cleaned up Redis connections before pod termination");
}
}
For Kubernetes deployments, configure proper termination grace periods:
apiVersion: apps/v1
kind: Deployment
metadata:
name: redis-client-app
spec:
template:
spec:
terminationGracePeriodSeconds: 30
containers:
- name: app
lifecycle:
preStop:
exec:
command: ["/bin/sh", "-c", "sleep 5"] # Allow time for connection cleanup
EKS Networking Concepts that Impact Redis Connectivity
Understanding how EKS networking works is crucial for troubleshooting Redis connectivity issues, as it involves multiple layers including the AWS VPC CNI plugin which provides pod networking, security group configurations that control access between pods and Redis endpoints, service discovery mechanisms for locating Redis instances, and proper subnet configurations to ensure pods can reach Redis clusters across availability zones.
AWS VPC CNI Overview
The Amazon VPC CNI plugin is the default networking solution for EKS and has several important characteristics:
- Each pod receives a real VPC IP address
- Pod IP addresses come from the node’s subnet
- IP address limits exist per node based on instance type
- Security groups from nodes apply to pod traffic
This architecture means your pods communicate directly with Redis without NAT, simplifying security configurations but requiring appropriate subnet and security group settings.
Pod Density and IP Address Exhaustion
EKS nodes have limits on the number of IP addresses they can utilize3:
Instance Type | Maximum Pod IPs |
---|---|
t3.small | 11 |
m5.large | 29 |
c5.xlarge | 58 |
If pods can’t get IP addresses, they remain in a Pending state, impacting application availability. Monitor IP address usage:
# Check available IP addresses per node
kubectl describe node | grep "Allocated"
Service Discovery Mechanisms
For accessing Redis services, here are common approaches:
1. Using External Name Services:
apiVersion: v1
kind: Service
metadata:
name: redis-master
spec:
type: ExternalName
externalName: my-redis.xxxx.ng.0001.use1.cache.amazonaws.com
2. Using environment variables and ConfigMaps:
apiVersion: v1
kind: ConfigMap
metadata:
name: redis-config
data:
redis-host: "my-redis.xxxx.ng.0001.use1.cache.amazonaws.com"
redis-port: "6379"
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: app
spec:
template:
spec:
containers:
- name: app
envFrom:
- configMapRef:
name: redis-config
Security Group Considerations
EKS pod traffic inherits the security group of the node. For ElastiCache connectivity:
- Identify node security groups:
aws eks describe-nodegroup --cluster-name your-cluster --nodegroup-name your-nodegroup --query 'nodegroup.remoteAccessSecurityGroup'
- Configure ElastiCache security groups:
aws elasticache modify-replication-group --security-group-ids sg-012345abcdef --replication-group-id your-redis-cluster
Monitoring and Observability Strategies
Implementing comprehensive monitoring helps detect Redis connectivity issues before they impact your applications.
Key Metrics to Monitor
For Redis:
- CurrConnections: Current client connections (alert on sudden changes)
- NetworkBytesIn/Out: Network traffic patterns
- CPUUtilization: CPU usage (spikes during command processing)
- SwapUsage: Indicates memory pressure
- CommandLatency: Response time for operations
For Client Applications:
- Connection timeouts and errors
- Connection pool utilization
- Request latency to Redis
- Retry counts and circuit breaker activations
CloudWatch Dashboard for Redis Monitoring
Create comprehensive dashboards for Redis monitoring:
aws cloudwatch put-dashboard --dashboard-name "Redis-Monitoring" --dashboard-body '{
"widgets": [
{
"type": "metric",
"x": 0,
"y": 0,
"width": 12,
"height": 6,
"properties": {
"metrics": [
[ "AWS/ElastiCache", "CurrConnections", "CacheClusterId", "my-redis" ]
],
"period": 60,
"stat": "Average",
"region": "us-east-1",
"title": "Current Connections"
}
},
{
"type": "metric",
"x": 12,
"y": 0,
"width": 12,
"height": 6,
"properties": {
"metrics": [
[ "AWS/ElastiCache", "CPUUtilization", "CacheClusterId", "my-redis" ]
],
"period": 60,
"stat": "Average",
"region": "us-east-1",
"title": "CPU Utilization"
}
}
]
}'
Prometheus and Grafana Integration
For more detailed monitoring, export Redis metrics to Prometheus:
apiVersion: v1
kind: ConfigMap
metadata:
name: redis-exporter-config
data:
redis-exporter.conf: |
redis.addr=my-redis:6379
namespace=redis
---
apiVersion: apps/v1
kind: Deployment
metadata:
name: redis-exporter
spec:
template:
spec:
containers:
- name: exporter
image: oliver006/redis_exporter:latest
ports:
- containerPort: 9121
envFrom:
- configMapRef:
name: redis-exporter-config
Distributed Tracing
Implement distributed tracing to identify Redis-related bottlenecks:
// Using Spring Cloud Sleuth with Redis
@Bean
public RedisTemplate<String, Object> redisTemplate(
RedisConnectionFactory redisConnectionFactory,
SpanCustomizer spanCustomizer) {
RedisTemplate<String, Object> template = new RedisTemplate<>();
template.setConnectionFactory(
new TracingRedisConnectionFactory(
redisConnectionFactory,
"redis",
spanCustomizer
)
);
return template;
}
Conclusion and Checklist
Redis connectivity issues in EKS environments can be complex but are solvable with a systematic approach. The combination of proper client configuration, container lifecycle management, and infrastructure sizing is key to reliable Redis operations in containerized environments.
Redis Connectivity Troubleshooting Checklist
Network Connectivity
- Verify DNS resolution to Redis endpoint
- Confirm security groups allow traffic from EKS nodes
- Test basic connectivity using nc, telnet, and redis-cli
- Verify proper routing between pod subnets and Redis
Client Configuration
- Use explicit connection pool settings instead of defaults
- Set appropriate timeouts for connection and operations
- Implement connection health checks
- Configure proper connection handling during pod termination
Redis Instance Sizing
- Ensure instance type can handle expected connection count
- Monitor memory usage and consider enabling maxmemory policy
- Evaluate read/write splitting using read replicas for read-heavy workloads
- Consider Redis Cluster for large datasets or high throughput
Scaling Behavior
- Implement gradual scaling policies in HPA configuration
- Monitor connection patterns during scale events
- Consider connection limiting at the application level
- Test application behavior during both scale-out and scale-in events
Monitoring and Alerting
- Set up CloudWatch alarms for Redis metrics (connections, CPU, memory)
- Implement application-level metrics for Redis operations
- Configure distributed tracing for end-to-end visibility
- Create dashboard showing correlated application and Redis metrics
By addressing these areas systematically, you can build reliable, scalable systems that maintain consistent performance even under peak loads. Remember that Redis connectivity in EKS is not just about the initial setup but about creating resilient systems that gracefully handle the dynamic nature of containerized environments.
References